Appearance
Exporting the embedded wallet
Privy enables your users to export the full private key for their embedded wallet.
This allows them to use their embedded wallet address with another wallet client, such as MetaMask in any application. For example, if a user purchases an asset in your app with their embedded wallet, they can still use that asset outside of your app by exporting their embedded wallet's private key to another wallet client.
To have your user export their embedded wallet's private key, use the exportWallet
method from the usePrivy
hook.
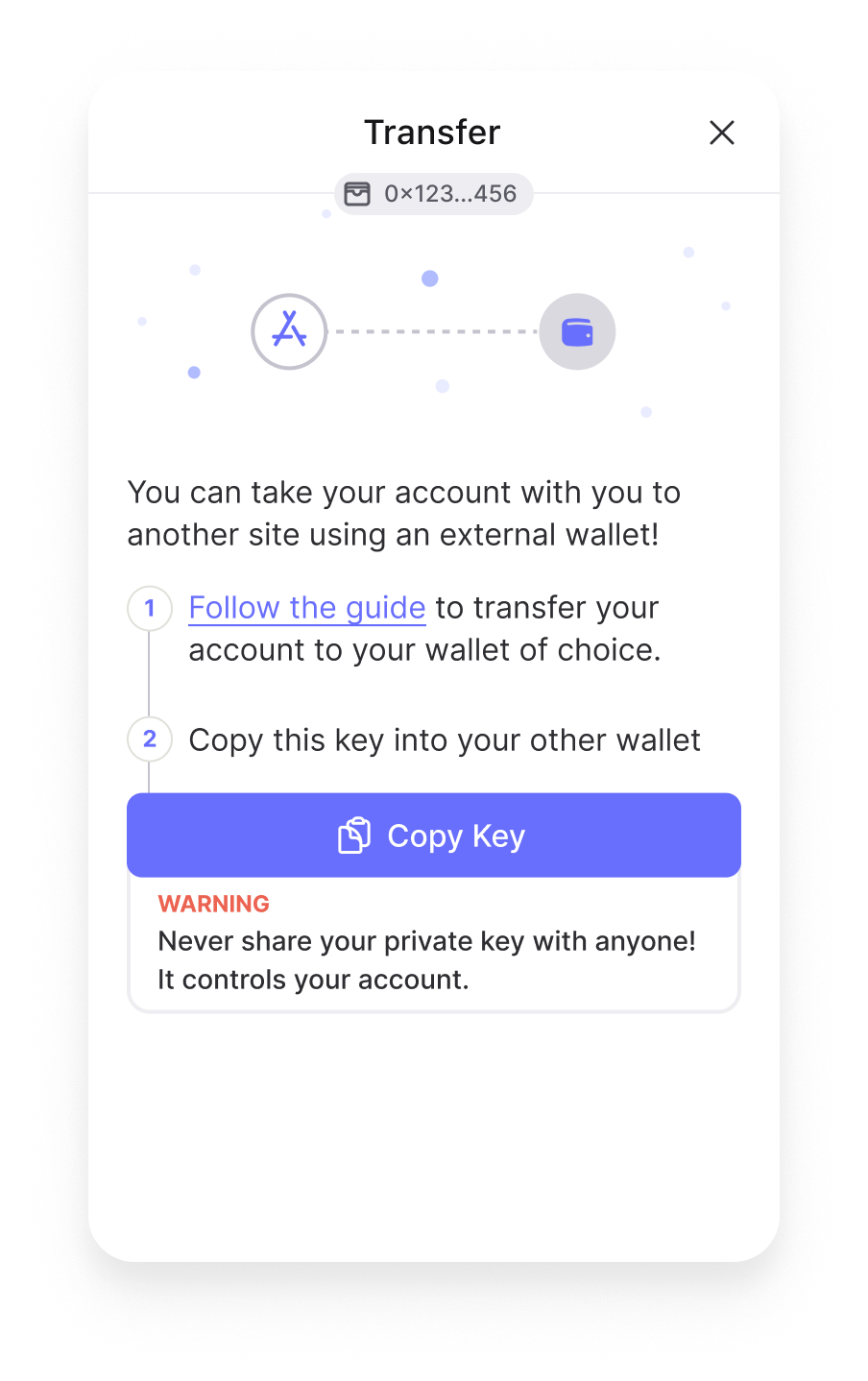
When invoked, exportWallet
will open a modal where your user can copy the full private key for their embedded wallet. The modal will also link your user to a guide for how to load their embedded wallet private key into another wallet client, such as MetaMask, Coinbase Wallet, or Rainbow.
If your user is not authenticated
or has not yet created an embedded wallet in your app, this method will fail.
As an example, you might attach exportWallet
as an event handler to an export wallet button in your app:
tsx
import {usePrivy} from '@privy-io/react-auth';
function ExportWalletButton() {
const {ready, authenticated, user, exportWallet} = usePrivy();
// Check that your user is authenticated
const isAuthenticated = ready && authenticated;
// Check that your user has an embedded wallet
const hasEmbeddedWallet = !!user.linkedAccounts.find(
(account) => account.type === 'wallet' && account.walletClient === 'privy',
);
return (
<button onClick={exportWallet} disabled={!isAuthenticated || !hasEmbeddedWallet}>
Export my wallet
</button>
);
}
Privy tip![]()
When your user exports their embedded wallet, their private key is assembled on a different origin than your app's origin. This means neither you nor Privy can ever access your user's private key. Your user is the only party that can ever access their full private key.