Appearance
Enrolling your users in wallet MFA ​
Once you have enabled MFA for your app, to prompt your users to enroll in MFA for their embedded wallet, use the showMfaEnrollmentModal
method from the useMfaEnrollment
hook.
tsx
import {useMfaEnrollment} from '@privy-io/react-auth';
function MfaEnrollmentButton() {
const {showMfaEnrollmentModal} = useMfaEnrollment();
return <button onClick={showMfaEnrollmentModal}>Enroll in MFA</button>;
}
When invoked, showMfaEnrollmentModal
will open a Privy modal that prompts the user to select their desired MFA method from the ones you've enabled for your app, and will guide them through the enrollment process.
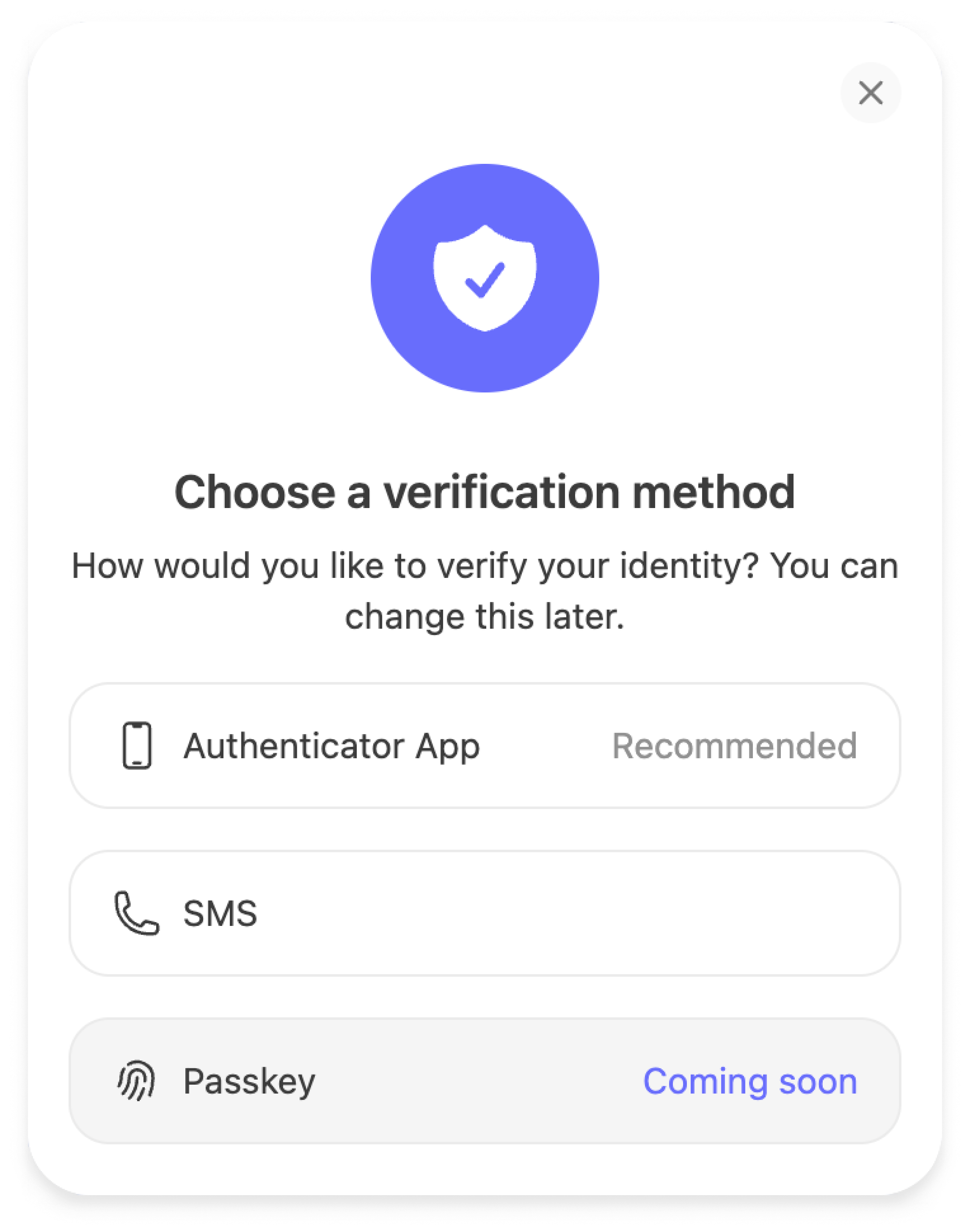
Once a user has successfully enrolled an MFA method, the user's enrolled method will appear under the mfaMethods
field of their user
object:
tsx
const {user} = usePrivy();
console.log(user.mfaMethods);
// ['sms', 'totp', 'passkey'] for a user who has enrolled in all of SMS, TOTP, and passkey MFA
Enrollment flows ​
The user flow for enrolling in each MFA method is outlined below:
SMS ​
Privy will prompt the user to enter their phone number and will send the user an SMS with a 6-digit MFA code. Users must then enter this code into the Privy modal to complete enrollment.
TOTP ​
Privy will show the user a QR code that they can scan with an authenticator app. Users must then enter the 6-digit MFA code provided by their authenticator app into the Privy modal to complete enrollment. If the user cannot easily scan the QR code from the modal (e.g. they accessing your app from a mobile browser), they may instead copy-paste the URI-encoded contents of the QR code into their authenticator app.
Passkey ​
Privy will enable any registered passkeys to be used for MFA. If the user has not yet registered a passkey with your app, they can select the "Passkey" option in Privy's MFA enrollment modal to do so.
Managing MFA methods ​
To allow your users to modify their MFA methods, simply invoke the showMfaEnrollmentModal
method from the useMfaEnrollment
hook. This is the same method you would use to prompt your user to enroll in MFA for the first time.
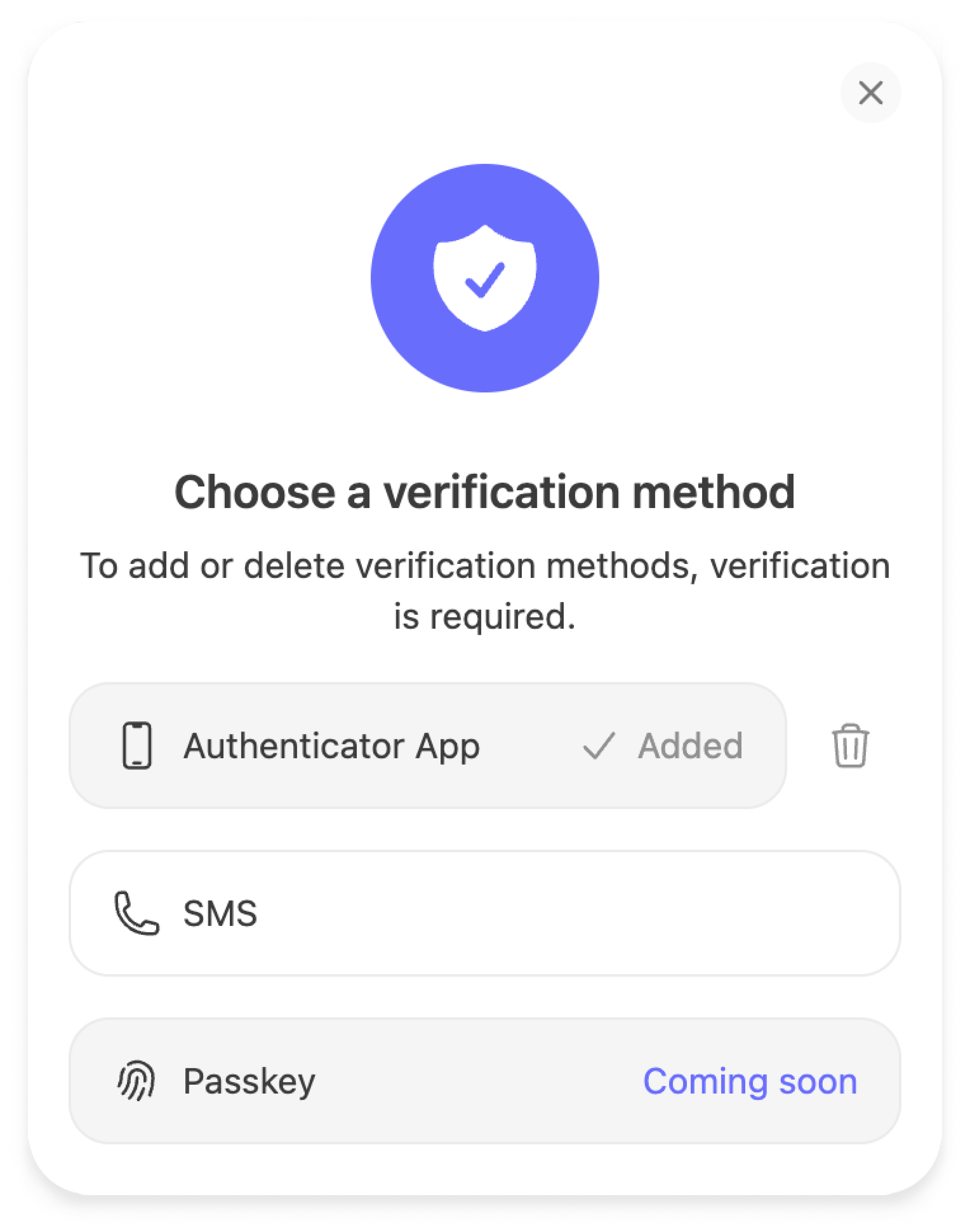
Within this modal, users can remove existing MFA methods or enroll in additional ones for their embedded wallet. Prior to making changes to their MFA methods, users will be prompted to re-verify their identity using one of their existing MFA methods.
INFO
By default, removing a passkey as MFA will also unlink it as a valid login method. In order to modify this behavior, you can set the shouldUnlinkOnUnenrollMfa
option to false
under the passkeys
config in PrivyProvider
.
tsx
<PrivyProvider
appId="your-privy-app-id"
config={{
...theRestOfYourConfig,
passkeys: {
// Set this to `false` if you wish to keep the passkey as a login method after unenrolling from MFA.
shouldUnlinkOnUnenrollMfa: false,
},
}}
>
{/* your app's content */}
</PrivyProvider>