Appearance
Password-based recovery
This guide explains how to set up passwords for user-managed recovery of embedded wallets.
Start with a walkthrough of the user experience of password-based recovery, and continue to concrete configuration details below.
User experience
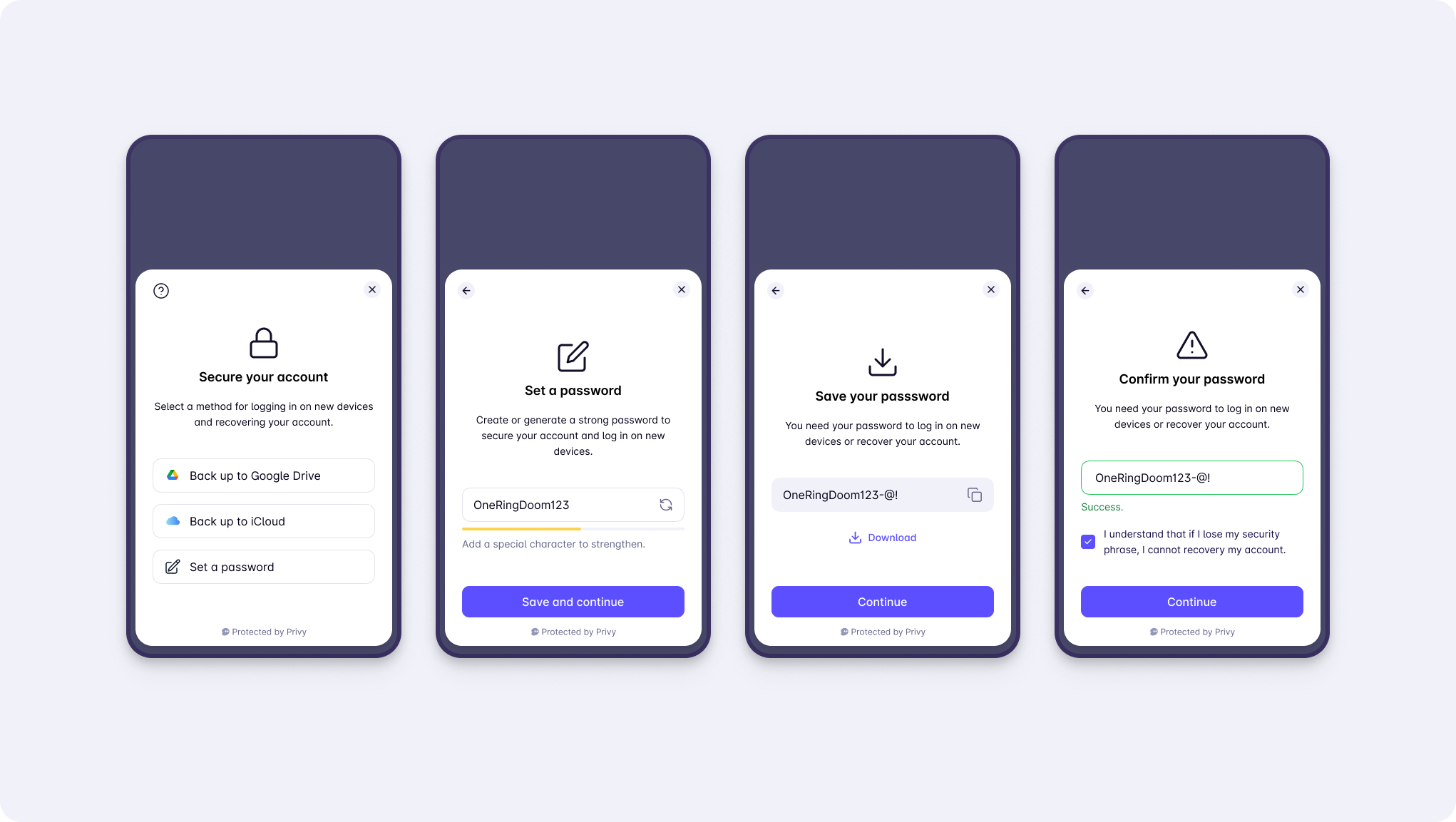
Setup
When a user is prompted to set a password for their recovery share, the Privy modal will open in your app and guide the user through setting a secure password for their wallet.
The modal will require that the user confirm their password and will stress the importance of not losing the password, as loss of the password may lead to loss of access to the wallet.
Recovery
Once a user has set a password, when they attempt to use the wallet on a new device or browser, Privy will automatically prompt the user to input their password to recover the wallet.
Once the user successfully inputs their password, they can use the wallet on that device, and will not be prompted for the password on that device again.
Integration
Configuration
There is nothing you need to do to configure passwords as an option for user-managed recovery. Passwords are always an option for user-managed recovery flows.
Prompting users to set a password
Your app can either prompt users to set a password when they first create their wallet, or at a later point in time.
When a user is prompted to set a password, the Privy modal will open and prompt the user to set a secure password for their wallet. The modal will require that the user confirm their password and will stress the importance of not losing the password, as loss of the password may lead to loss of access to the wallet.
Requiring a password on wallet creation
To require that users set a password when they first create their wallet, visit the Embedded wallets page of the Privy Dashboard and navigate to the Recovery methods tab. Toggle the Require recovery method on wallet creation option on.
When this setting is on, users will automatically be prompted to set up user-managed recovery when they create their wallet. Note that:
- When setting up user-managed recovery, users can choose any of the recovery methods you have enabled in the Dashboard. This will always include passwords.
- If the user does not successfully set a password (or any other enabled user-managed recovery factor) when creating their wallet, a wallet will not be created for them.
Setting a password at a later point
To have users set a password for a wallet after they have created it, or to reset their password, see our guide for recovery upgrade.